Python Set Operations Explained: From Theory to Real-Time Applications
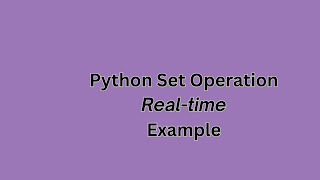
This lambda function sorts a list of tuples based on the second element of each tuple.
python code
my_list = [(1, 2), (4, 1), (9, 10), (13, 6), (5, 7)]
sorted_list = sorted(my_list, key=lambda x: x[1])
print(sorted_list)
Output:
[(4, 1), (1, 2), (13, 6), (5, 7), (9, 10)]
** Process exited - Return Code: 0 **
Press Enter to exit terminal
This lambda function finds the maximum value in a list of dictionaries based on a specific key.
python code
my_list = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 20}]
max_age = max(my_list, key=lambda x: x['age'])
print(max_age)
Output:
{'name': 'Bob', 'age': 30}
** Process exited - Return Code: 0 **
Press Enter to exit terminal
This lambda function multiplies two lists element-wise and returns the result as a new list.
python code
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
result = list(map(lambda x, y: x * y, list1, list2))
print(result)
Output:
[5, 12, 21, 32]
** Process exited - Return Code: 0 **
Press Enter to exit terminal
This lambda function filters a list of integers and returns only the even numbers.
python code
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, my_list))
print(even_numbers)
Output:
[2, 4, 6, 8, 10]
** Process exited - Return Code: 0 **
Press Enter to exit terminal
This lambda function creates a function that returns another function that adds a constant value to its input.
python code
def add_constant(const):
return lambda x: x + const
add5 = add_constant(5)
add10 = add_constant(10)
print(add5(3))
print(add10(3))
Output:
8
13
** Process exited - Return Code: 0 **
Press Enter to exit terminal
In the above example, add_constant is a function that returns a lambda function that adds the constant value to its input. add5 and add10 are two different functions that add 5 and 10 to their input, respectively.
Related
Comments
Post a Comment
Thanks for your message. We will get back you.