Python Set Operations Explained: From Theory to Real-Time Applications
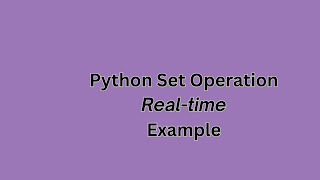
In Pandas, bfill and ffill are two important methods used for filling missing values in a DataFrame or Series by propagating the previous (forward fill) or next (backward fill) valid values respectively. These methods are particularly useful when dealing with time series data or other ordered data where missing values need to be filled based on the available adjacent values.
When you use the ffill method on a DataFrame or Series, it fills missing values with the previous non-null value in the same column. It propagates the last known value forward.
This method is often used to carry forward the last observed value for a specific column, making it a good choice for time series data when the assumption is that the value doesn't change abruptly.
Example:
import pandas as pd
data = {'A': [1, 2, None, 4, None, 6],
'B': [None, 'X', 'Y', None, 'Z', 'W']}
df = pd.DataFrame(data)
print(df)
# Output:
# A B
# 0 1.0 None
# 1 2.0 X
# 2 NaN Y
# 3 4.0 None
# 4 NaN Z
# 5 6.0 W
# Forward fill missing values
df_filled = df.ffill()
print(df_filled)
# Output:
# A B
# 0 1.0 None
# 1 2.0 X
# 2 2.0 Y
# 3 4.0 Y
# 4 4.0 Z
# 5 6.0 W
The bfill method fills missing values with the next non-null value in the same column. It propagates the next known value backward.
Like ffill, this method can be useful in time series data, especially when values are missing at the beginning of the dataset.
Example:
import pandas as pd
data = {'A': [None, 2, None, 4, 5, None],
'B': ['X', 'Y', None, None, 'Z', None]}
df = pd.DataFrame(data)
print(df)
# Output:
# A B
# 0 NaN X
# 1 2.0 Y
# 2 NaN None
# 3 4.0 None
# 4 5.0 Z
# 5 NaN None
# Backward fill missing values
df_filled = df.bfill()
print(df_filled)
# Output:
# A B
# 0 2.0 X
# 1 2.0 Y
# 2 4.0 None
# 3 4.0 Z
# 4 5.0 Z
# 5 NaN None
These methods can be very useful for handling missing data when the order of the data is important, such as in time series analysis.
Comments
Post a Comment
Thanks for your message. We will get back you.