Python Set Operations Explained: From Theory to Real-Time Applications
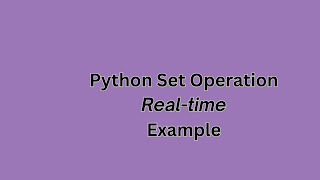
Creating a complex Python script is challenging, but I can provide you with a simplified example of a script that simulates a basic bank account system. In a real-world application, this would be much more elaborate, but here's a concise version.
Here is an example of a Python script that explains each step:
class BankAccount:
def __init__(self, account_holder, initial_balance=0):
self.account_holder = account_holder
self.balance = initial_balance
def deposit(self, amount):
if amount > 0:
self.balance += amount
print(f"Deposited ${amount}. New balance: ${self.balance}")
else:
print("Invalid deposit amount.")
def withdraw(self, amount):
if 0 < amount <= self.balance:
self.balance -= amount
print(f"Withdrew ${amount}. New balance: ${self.balance}")
else:
print("Invalid withdrawal amount or insufficient funds.")
def get_balance(self):
print(f"Account balance for {self.account_holder}: ${self.balance}")
# Example usage:
if __name__ == "__main__":
account1 = BankAccount("Alice", 1000)
account2 = BankAccount("Bob")
account1.deposit(500)
account2.deposit(750)
account1.withdraw(200)
account2.withdraw(1000)
account1.get_balance()
account2.get_balance()
This script defines a BankAccount class with methods for depositing, withdrawing, and checking the balance. In the example usage section, two bank accounts are created for Alice and Bob, and various transactions are made.
Please note that this is a simplified example for demonstration purposes. In a real banking system, you would need more robust security measures, data persistence, and error handling. Additionally, the code would typically be spread across multiple files for better organization and maintainability.
Related
Comments
Post a Comment
Thanks for your message. We will get back you.